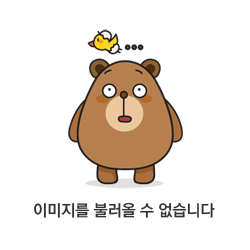
import java.util.*;
class Program {
// Do not edit the class below except
// for the depthFirstSearch method.
// Feel free to add new properties
// and methods to the class.
static class Node {
String name;
List<Node> children = new ArrayList<Node>();
public Node(String name) {
this.name = name;
}
public List<String> depthFirstSearch(List<String> array) {
array.add(name);
for (Node child : children){
if (child == null){
return array;
}
child.depthFirstSearch(array);
}
return array;
}
public Node addChild(String name) {
Node child = new Node(name);
children.add(child);
return this;
}
}
}
'Algorithms and Data Structures > Coding Practices' 카테고리의 다른 글
AlgoExpert Class Photos (0) | 2022.07.13 |
---|---|
AlgoExpert Minimum Waiting Time (0) | 2022.07.12 |
AlgoExpert Node Depths (0) | 2022.07.12 |
AlgoExpert Sorted Squared Array (0) | 2022.07.10 |
AlgoExpert Non-Constructible Change (0) | 2022.07.10 |